
As usual, we need to deal with some data(No, Name, Age, Mob No, Address). So, we need to create the model of the data and implement that model into the sqlite db of django. To do so, look for the file models.py in your app folder and add the code below:-from django.db import models # Create your models here. At this point of our tutorial, we've a mysql database for persisting data and created a virtual environment for installing the project packages. Django 3 Tutorial, Step 3 - Installing Django and MySQL Client. In this step, we'll install django and mysql client from PyPI using pip in our activated virtual environment.
Deploying Django with Apache and mod_wsgi is a tried and tested way to getDjango into production.

mod_wsgi is an Apache module which can host any Python WSGI application,including Django. Django will work with any version of Apache which supportsmod_wsgi.
The official mod_wsgi documentation is your source for all the details abouthow to use mod_wsgi. You'll probably want to start with the installation andconfiguration documentation.
Basic configuration¶
Once you've got mod_wsgi installed and activated, edit your Apache server'shttpd.conf file and add the following.
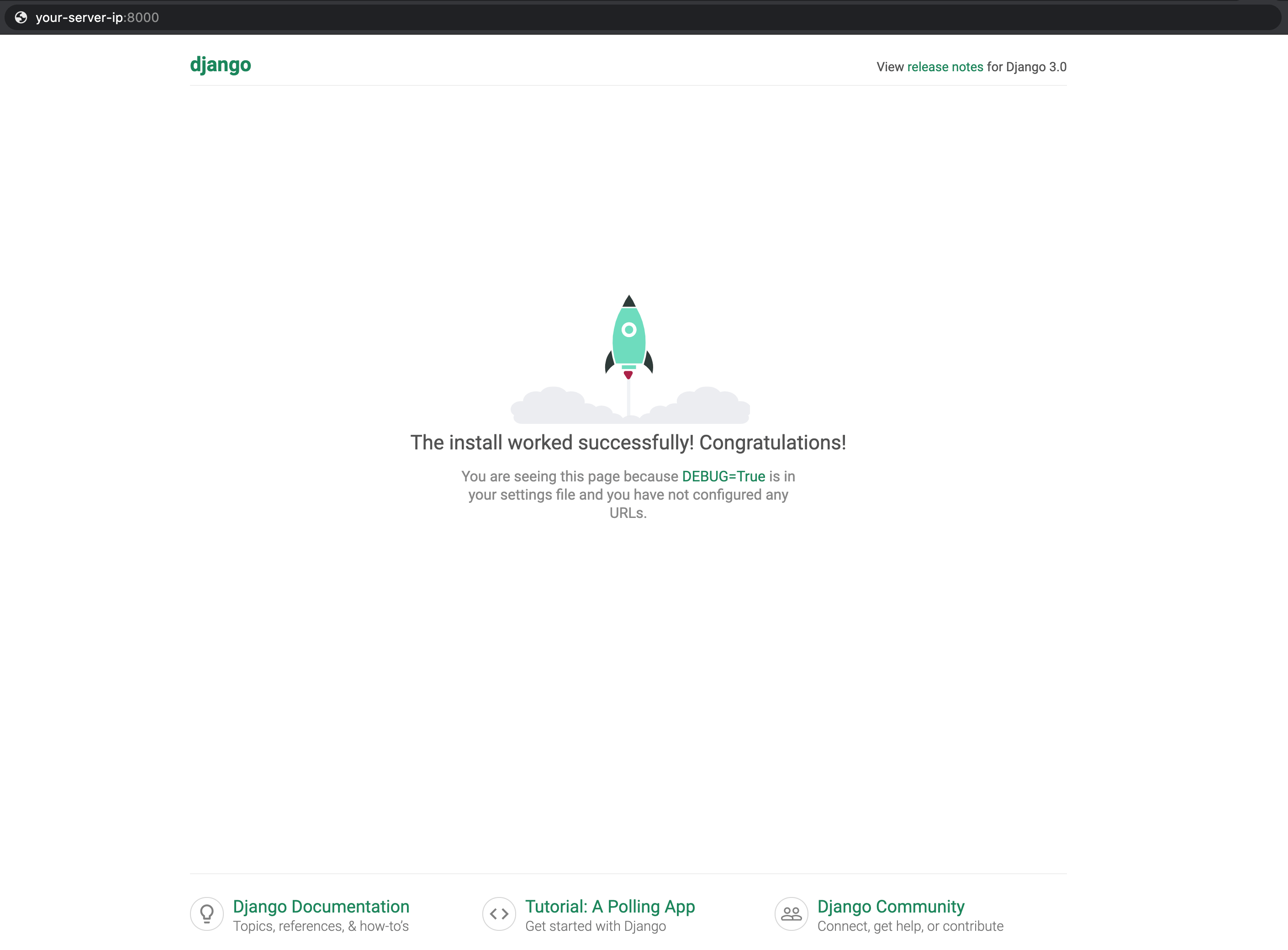
As usual, we need to deal with some data(No, Name, Age, Mob No, Address). So, we need to create the model of the data and implement that model into the sqlite db of django. To do so, look for the file models.py in your app folder and add the code below:-from django.db import models # Create your models here. At this point of our tutorial, we've a mysql database for persisting data and created a virtual environment for installing the project packages. Django 3 Tutorial, Step 3 - Installing Django and MySQL Client. In this step, we'll install django and mysql client from PyPI using pip in our activated virtual environment.
Deploying Django with Apache and mod_wsgi is a tried and tested way to getDjango into production.
mod_wsgi is an Apache module which can host any Python WSGI application,including Django. Django will work with any version of Apache which supportsmod_wsgi.
The official mod_wsgi documentation is your source for all the details abouthow to use mod_wsgi. You'll probably want to start with the installation andconfiguration documentation.
Basic configuration¶
Once you've got mod_wsgi installed and activated, edit your Apache server'shttpd.conf file and add the following.
The first bit in the WSGIScriptAlias
line is the base URL path you want toserve your application at (/
indicates the root url), and the second is thelocation of a 'WSGI file' – see below – on your system, usually inside ofyour project package (mysite
in this example). This tells Apache to serveany request below the given URL using the WSGI application defined in thatfile.
If you install your project's Python dependencies inside a virtualenvironment
, add the path using WSGIPythonHome
. See the mod_wsgivirtual environment guide for more details.
The WSGIPythonPath
line ensures that your project package is available forimport on the Python path; in other words, that importmysite
works.
The piece ensures that Apache can access your
wsgi.py
file.
Next we'll need to ensure this wsgi.py
with a WSGI application objectexists. As of Django version 1.4, startproject
will have created onefor you; otherwise, you'll need to create it. See the WSGI overviewdocumentation for the default contents youshould put in this file, and what else you can add to it.
Warning
If multiple Django sites are run in a single mod_wsgi process, all of themwill use the settings of whichever one happens to run first. This can besolved by changing:
in wsgi.py
, to:
or by using mod_wsgi daemon mode and ensuring that eachsite runs in its own daemon process.
Fixing UnicodeEncodeError
for file uploads
If you get a UnicodeEncodeError
when uploading files with file namesthat contain non-ASCII characters, make sure Apache is configured to acceptnon-ASCII file names:
A common location to put this configuration is /etc/apache2/envvars
.
See the Files section of the Unicode reference guide fordetails.
Using mod_wsgi
daemon mode¶
'Daemon mode' is the recommended mode for running mod_wsgi (on non-Windowsplatforms). To create the required daemon process group and delegate theDjango instance to run in it, you will need to add appropriateWSGIDaemonProcess
and WSGIProcessGroup
directives. A further changerequired to the above configuration if you use daemon mode is that you can'tuse WSGIPythonPath
; instead you should use the python-path
option toWSGIDaemonProcess
, for example:
If you want to serve your project in a subdirectory(https://example.com/mysite
in this example), you can add WSGIScriptAlias
to the configuration above:
See the official mod_wsgi documentation for details on setting up daemonmode.
Serving files¶
Django doesn't serve files itself; it leaves that job to whichever Webserver you choose.
We recommend using a separate Web server – i.e., one that's not also runningDjango – for serving media. Here are some good choices:
Django Xampp Mysql Login
- A stripped-down version of Apache
If, however, you have no option but to serve media files on the same ApacheVirtualHost
as Django, you can set up Apache to serve some URLs asstatic media, and others using the mod_wsgi interface to Django.
This example sets up Django at the site root, but serves robots.txt
,favicon.ico
, and anything in the /static/
and /media/
URL space asa static file. All other URLs will be served using mod_wsgi:
Django Xampp Mysql Free
Serving the admin files¶
When django.contrib.staticfiles
is in INSTALLED_APPS
, theDjango development server automatically serves the static files of theadmin app (and any other installed apps). This is however not the case when youuse any other server arrangement. You're responsible for setting up Apache, orwhichever Web server you're using, to serve the admin files.
Django Xampp Mysql Download
The admin files live in (django/contrib/admin/static/admin
) of theDjango distribution.
We strongly recommend using django.contrib.staticfiles
to handle theadmin files (along with a Web server as outlined in the previous section; thismeans using the collectstatic
management command to collect thestatic files in STATIC_ROOT
, and then configuring your Web server toserve STATIC_ROOT
at STATIC_URL
), but here are threeother approaches:
- Create a symbolic link to the admin static files from within yourdocument root (this may require
+FollowSymLinks
in your Apacheconfiguration). - Use an
Alias
directive, as demonstrated above, to alias the appropriateURL (probablySTATIC_URL
+admin/
) to the actual location ofthe admin files. - Copy the admin static files so that they live within your Apachedocument root.
Authenticating against Django's user database from Apache¶
Django provides a handler to allow Apache to authenticate users directlyagainst Django's authentication backends. See the mod_wsgi authenticationdocumentation.